Let's deploy our frontend app (that currently lives on localhost) to the Internet.
First, let's build the app! Go to frontend directory and run:
- yarn run build
We'll be using a new s3 bucket to host our frontend app! Add the new bucket to the stack file:
const websiteBucket = new s3.Bucket(this, "WebsiteBucket", {// so that it's publicly availablepublicReadAccess: true,// the index documentwebsiteIndexDocument: "index.html"});
Instead of copying the contents of the build folder manually, let's deploy it automatically by adding this:
new s3Deployment.BucketDeployment(this, "DeployWebsite", {destinationBucket: websiteBucket,// path to our build directorysources: [s3Deployment.Source.asset("../frontend/build")]});
Lastly, just like with the logo url, let's output the website url.
new cdk.CfnOutput(this, "WebsiteUrl", {value: websiteBucket.bucketWebsiteUrl});
Deploy a site with HTTPS support behind a CDN with CDK
We want our website to be secure and served on https (and not on http) - let's fix that.
Also, in the previous lesson, we had to type out the three steps for deploying our frontend:
- Creating a bucket
- Creating a bucket deployment
- Creating a CloudFormation output
We can solve both things by using CDK-SPA-Deploy! It can be used to deploy an SPA (Single Page Application), written in reactJS (or Angular/Vue) to aws behind SSL (meaning https) on CloudFront.
To add it, run:
- npm install --save cdk-spa-deploy
🤔 Cloudfront is Amazon's CDN (Content Delivery Network).
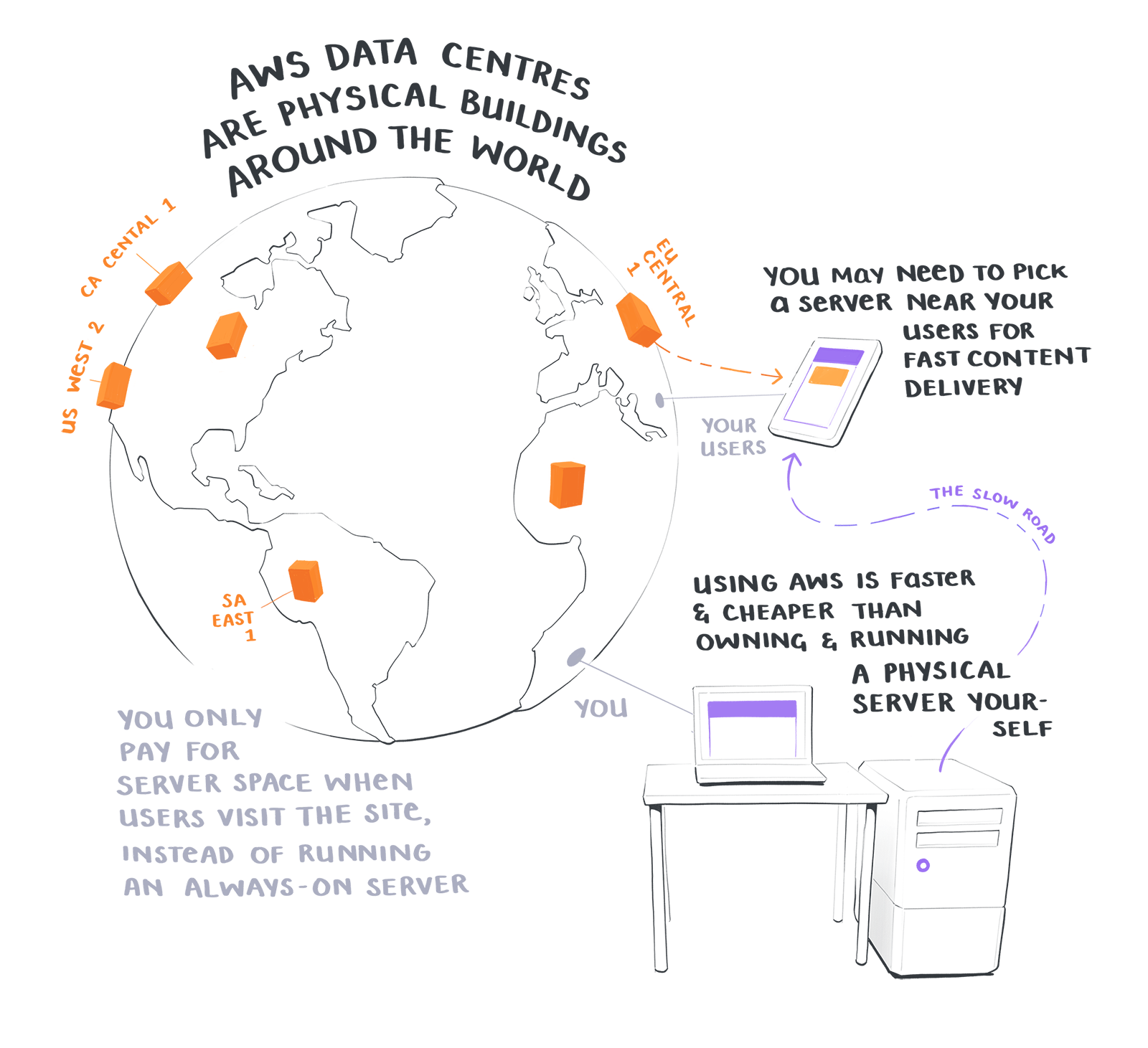
Import it to the stack file:
- import { SPADeploy } from "cdk-spa-deploy";
Then comment out (or remove) the three sections that we wrote in the last time lesson and replace them with:
new SPADeploy(this, "WebsiteDeployment").createSiteWithCloudfront({indexDoc: "index.html",websiteFolder: "../frontend/build"});